Code Snippets
These are bits of code taken from working applications that do something useful. Although they worked in the original applications, they could be slightly modified and placed here without any specific testing and out of context - use it at your own risk.
Common C/C++
Log formatted message with timestamp to file
Tree-like text command structure parsing
Trim std::string
Simple C++ callback
Another C++ singleton template
RC4 symmetric file encrypt/decrypt
Get offset value in bytes of member in the structure type
Limit variable value to permitted range
Wildcard string matching
Compile-time error checking
Circular FIFO template
DevCpp: Blowfish algorithm test (including required OpenSSL files)
WinAPI specific
Measure time taken by execution of some code part
Get module handle to DLL from inside DLL without knowing DLL name
Get executable file version
Show info about last error
Detect windows suspend/hibernate (S3/S4)
Win32 mutex & scoped lock
Run screensaver, turn off monitor
Find window at cursor
Flashing scroll lock
Sending key events to specified window
VCL specific
Animated tray icon class
C++ Callbacks using Borland's __closure
Add animation as resource (Borland)
Autosize report style TListView's column
Virtual vs common TListView
Using Indy with free Turbo C++ Explorer
Iterate through TPanel's childs
Catching messages with VCL using overriden WndProc
Catching messages for another component (VCL)
Wildcard matching VCL way
Custom shape form
Zlib test
C++/CLI
Log formatted message with timestamp to file
#include <stdio.h> #include <time.h> #include <sys\timeb.h> //usage: logtxt("log test %d\r\n", 1234); void logtxt(char *lpData, ...) { FILE* fout; va_list ap; char buf[1024]; //determines max message length struct timeb timebuffer; char *timeline; ftime( &timebuffer ); timeline = ctime( & ( timebuffer.time ) ); timeline[19]=0; sprintf(buf, "%8s.%03hu: ", &timeline[11], timebuffer.millitm); va_start(ap, lpData); vsnprintf(buf + strlen(buf), 1024-strlen(buf), lpData, ap); va_end(ap); buf[sizeof(buf) - 1] = '\0'; if (fout = fopen("myapp.log","at+")) { fwrite(buf, strlen(buf), 1, fout); fclose(fout); } }
Measure time taken by execution of some code part
LARGE_INTEGER m_StartCounter, m_EndCounter, Freq; __int64 m_ElapsedTime, *F; if (!QueryPerformanceFrequency(&Freq)) { ShowMessage("QueryPerformanceFrequency failed"); //VCL return; } F=(__int64*)&Freq; QueryPerformanceCounter (&m_StartCounter); ////////////////////////////// // do some operations to check ////////////////////////////// QueryPerformanceCounter (&m_EndCounter); m_ElapsedTime = (m_EndCounter.QuadPart - m_StartCounter.QuadPart ); ShowMessage("Execution time: " + FloatToStrF((double)m_ElapsedTime/(double)*F, ffGeneral, 5, 5)); //VCL
Tree-like text command structure parsing
/* Usage: if (StartsWith(szMsg, "get ")) { if (StartsWith(szMsg, "status ")) { if (StartsWith(szMsg, "mode ")) { //... } else if (StartsWith(szMsg, "description ")) { SetStatusDesc(szMsg); } } } */ inline bool StartsWith(char* &a, char *b) { if(strlen(a) < strlen(b)) { return false; } else { bool result = !strnicmp(a,b,strlen(b)); //case insensitive if(result) a += strlen(b); return result; } }
Trim std::string
inline std::string TrimStr(const std::string& src, const std::string& c = " \r\n") { int stop = src.find_last_not_of(c); if (stop == std::string::npos) return std::string(); int start = src.find_first_not_of(c); if (start == std::string::npos) start = 0; return src.substr(start, (stop-start)+1); }
Get module handle to DLL from inside DLL without knowing DLL name
//useful for creating plugins using WinAPI when DLLMain() is unavailable HMODULE GetCurrentModule() { MEMORY_BASIC_INFORMATION mbi; static int dummy; VirtualQuery( &dummy, &mbi, sizeof(mbi) ); return reinterpret_cast<HMODULE>(mbi.AllocationBase); }
Get executable file version (VCL)
//i.e. GetFileVersion(Application->ExeName) AnsiString GetFileVersion(AnsiString FileName) { AnsiString asVer=""; VS_FIXEDFILEINFO *pVsInfo; unsigned int iFileInfoSize = sizeof( VS_FIXEDFILEINFO ); int iVerInfoSize = GetFileVersionInfoSize(FileName.c_str(), NULL); if (iVerInfoSize!= 0) { char *pBuf = new char[iVerInfoSize]; if (GetFileVersionInfo(FileName.c_str(),0, iVerInfoSize, pBuf ) ) { if (VerQueryValue(pBuf, "\\",(void **)&pVsInfo,&iFileInfoSize)) { asVer = IntToStr( HIWORD(pVsInfo->dwFileVersionMS) )+"."; asVer += IntToStr( LOWORD(pVsInfo->dwFileVersionMS) )+"."; asVer += IntToStr( HIWORD(pVsInfo->dwFileVersionLS) )+"."; asVer += IntToStr( LOWORD(pVsInfo->dwFileVersionLS) ); } } delete [] pBuf; } return asVer; }
Show info about last error (WinAPI)
LPVOID lpMsgBuf; DWORD dw = GetLastError(); FormatMessage( FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM | FORMAT_MESSAGE_IGNORE_INSERTS, NULL, dw, MAKELANGID(LANG_NEUTRAL, SUBLANG_DEFAULT), (LPTSTR) &lpMsgBuf, 0, NULL ); MessageBox(NULL, (LPCTSTR)lpMsgBuf, TEXT("Error"), MB_OK); LocalFree(lpMsgBuf);
Simple C++ callback
It's not a snippet but a small Turbo C++ project, but I want to have it at hand.
Callback class/template is taken from
codeguru.
callbacks.zip
Animated tray icon class
Small Turbo C++ (BDS 2006) test project with animated tray icon. Additional features comparing to standard TTrayIcon:
- animation: icon list is passed using TImageList,
- recreation after shell restart (WM_TASKBARCREATE).
It uses some Borland-specific code (TImageList, TTimer, TNotifyEvent, FreeNotification),
so it will be unusable with non-VCL environments.
trayicon_test.zip
C++ Callbacks using Borland's __closure
Caller class: private: typedef void (__closure *CallbackClick)(void); public: CallbackClick callbackClick; .cpp (constructor) callbackClick = NULL; .cpp (execute callback): if (callbackClick) callbackClick(); Callee class: public: void CallbackClickFn(void); .cpp (init callback): caller->callbackClick = CallbackClickFn;
Detect windows suspend/hibernate (S3/S4)
.h: protected: void __fastcall WMPowerBroadcast(TMessage &Msg); BEGIN_MESSAGE_MAP VCL_MESSAGE_HANDLER(WM_POWERBROADCAST, TMessage, WMPowerBroadcast) END_MESSAGE_MAP(TForm) .cpp: void __fastcall TMainForm::WMPowerBroadcast(TMessage &Msg) { switch(Msg.WParam) { // not sended by Vista! //case PBT_APMQUERYSUSPEND: // MessageBeep(MB_OK); // break; case PBT_APMSUSPEND: // sended by Vista, // timeout = 2s only! S3Suspended = true; // do sth break; case PBT_APMQUERYSUSPENDFAILED: case PBT_APMRESUMESUSPEND: S3Suspended = false; // do sth break; } Msg.Result = 1; }
Another C++ singleton template
Win32 mutex & scoped lock
Run win32 screensaver
SendMessage(Application->Handle, WM_SYSCOMMAND, SC_SCREENSAVE, 0); //1->standby, 2->OFF SendMessage(Application->Handle, WM_SYSCOMMAND, SC_MONITORPOWER, 1);
Find window at cursor
// tested with Code::Blocks #include <iostream> #include <windows.h> using namespace std; int main() { for (int i=0; i<50; i++) { POINT pt; char caption[100]; GetCursorPos(&pt); HWND hWnd = WindowFromPoint(pt); GetWindowText(hWnd, caption, sizeof(caption)); cout << "Cursor position: " << pt.x << "/" << pt.y << ", caption: " << caption << endl; Sleep(1000); } return 0; }
Flashing scroll lock
// simulating key press // note: http://msdn.microsoft.com/en-us/library/ms645540(VS.85).aspx - codes keybd_event(VK_SCROLL, 0x45, KEYEVENTF_EXTENDEDKEY, 0); //down keybd_event(VK_SCROLL, 0x45, KEYEVENTF_EXTENDEDKEY | KEYEVENTF_KEYUP, 0); //upor
#include <WinAble.h> INPUT input[2]; //2 events sent in one call memset(&input, 0, sizeof(input)); input[0].type = input[1].type = INPUT_KEYBOARD; input[0].ki.wVk = input[1].ki.wVk = VK_SCROLL; input[1].ki.dwFlags = KEYEVENTF_KEYUP; //UINT SendInput(UINT nInputs, LPINPUT pInputs, int cbSize); //returns the number of events that it successfully inserted //into the keyboard or mouse input stream ::SendInput(2, input, sizeof(INPUT));
Sending key events to specified window
SendMessage(hWnd, WM_KEYDOWN, VK_RETURN, 0); SendMessage(hWnd, WM_KEYUP, VK_RETURN, 0);
RC4 symmetric file encrypt/decrypt
C++, BCB6 FileEncryptDecrypt.zip
Turbo C++, slightly more practical: FileEncryptDecrypt.zip
Note that RC4 uses XOR and encryption and decryption is exactly the same operation (application would encrypt file
if it is currently unencrypted and decrypt if it is encrypted and proper password is supplied). This is plain,
unmodified variant of RC4 and it should not be considered secure (similar to WEP).
Add animation as resource (Borland)
Create .rc file with line:
resourcename AVI filename
e.g. MYAVIFILE AVI C:\MyAvile.avi.
Add .rc file to project. At runtime set TAnimate's ResName:
e.g. Animate1->ResName = "MYAVIFILE";
Autosize report style TListView's column
//with object inspector: ListView1->Columns->Items[n]->AutoSize = true; //when adding/deleting items: ListView1->Width--; ListView1->Width++;
Virtual vs common TListView speed comparison
... or why not to use non-OwnerData TListView for large data collections: ownerdata_test.7z.
Get offset value in bytes of member in the structure type
#include <stddef.h>
int offset = offsetof (struct_type, member);
More at www.embedded.com
Limit variable value to permitted range
template<class T> inline T constrain(const T& value, const T& low, const T& high) { return (high < value) ? high : (value < low) ? low : value; } x = constrain(x, 1, 10);
Wildcard string matching
bool MatchPattern(char *element, char *pattern) { if( 0 == StrComp(pattern, "*") ) return true; if( (*element == '\0') && (*pattern != '\0') ) return false; if( *element == '\0' ) return true; switch( *pattern ) { case '*': if( MatchPattern(element, &pattern[1]) ) return true; else return MatchPattern(&element[1], pattern); case '?': return MatchPattern(&element[1], &pattern[1]); } if( *element == *pattern ) return MatchPattern(&element[1], &pattern[1]); else return false; }
Compile-time error checking
Circular FIFO template
CircularFifo.h
Another one: Fifo.h
DevCpp: simple Blowfish encryption and decryption test (including required OpenSSL files)
Using Indy with free Turbo C++ Explorer
#include <IdHTTP.hpp> //or other required
#pragma link "indy.lib" //for static build
TIdHTTP *IdHTTP = new TIdHTTP(NULL);
// etc...
indy_test.zip
Iterate through TPanel's childs
TGraphicControl* pGControl; TWinControl* pWControl; for (int i=0; i<Panel1->ControlCount; i++) { pGControl = dynamic_cast<TGraphicControl*>(Panel1->Controls[i]); pWControl = dynamic_cast<TWinControl*>(Panel1->Controls[i]); if (pGControl) { //... } if (pWControl) { //... } }
Catching messages using VCL message map
class TForm1 : public TForm { ... void __fastcall WmMove(TMessage& Msg); ... BEGIN_MESSAGE_MAP MESSAGE_HANDLER( WM_MOVE,TMessage,WmMove ) END_MESSAGE_MAP(TForm) ... }; void __fastcall TForm1::WmMove (TMessage& Msg) { ... // remove to block default processing TForm::Dispatch(&Msg); // Default processing ... }
Catching messages with VCL using overriden WndProc
class TForm1 : public TForm { ... virtual void __fastcall WndProc(Messages::TMessage &Message); ... }; void __fastcall TForm1::WndProc(Messages::TMessage &Message) { if (Message.Msg == WM_MOVE) { ... } TForm::WndProc(Message); // Default processing for any other message }
Catching messages for another component (VCL)
class TForm1 : public TForm { __fastcall TForm1 (TComponent* Owner) ; ... int (__stdcall *OldHookComponentProc) (); int (__stdcall *NewHookComponentProc) (); void __fastcall HookComponentProc(TMessage &aMsg); }; __fastcall TForm1::TForm1 (TComponent* _Owner): NewHookComponentProc (NULL) {} // hook Memo1: OldHookComponentProc = (int (__stdcall *)()) GetWindowLong(Memo1->Handle, GWL_WNDPROC); NewHookComponentProc = (int (__stdcall *)()) MakeObjectInstance(HookComponentProc); SetWindowLong(OwnerHandle, GWL_WNDPROC, (long) NewHookComponentProc); void __fastcall TForm1::HookComponentProc(TMessage &aMsg) { switch (aMsg.Msg) { case WM_SIZE: ... } // Default handler aMsg.Result = CallWindowProc(OldHookFormProc, OwnerHandle, aMsg.Msg, aMsg.WParam, aMsg.LParam); } // unhook Memo1: SetWindowLong(OwnerHandle, GWL_WNDPROC, (long)OldHookComponentProc); if (NewComponentFormProc) FreeObjectInstance(NewComponentFormProc); NewHookComponentProc = NULL;
Wildcard matching VCL way
// #include "masks.hpp" // #include <memory> bool bMatch; std::auto_ptr<TMask> foobarMask(new TMask("t?st*1")); bMatch = foobarMask->Matches("test1"); // true bMatch = foobarMask->Matches("tost11"); // true bMatch = foobarMask->Matches("tst1"); // false
Custom shape form
Nice looking hexagonal grid context menu with mouse over effect.
Made for miniscope v3 application. Used image is black and white grid
with emboss effect added using Paint.NET. Turn off transparency if you're planning to use this with Windows 98
or link setLayeredWindowAttributes dynamically.
custom_shape.zip
// except from FreeGG void TMainForm::SetTransparentWindow(int opacity) { typedef DWORD (WINAPI *FWINLAYER) (HWND hwnd,DWORD crKey,BYTE bAlpha,DWORD dwFlags); HINSTANCE hDll = LoadLibrary("user32.dll"); FWINLAYER setLayeredWindowAttributes = (FWINLAYER)GetProcAddress(hDll,"SetLayeredWindowAttributes"); if(setLayeredWindowAttributes != NULL) { SetWindowLong(this->Handle, GWL_EXSTYLE, WS_EX_LAYERED); setLayeredWindowAttributes(this->Handle, 0, 255-opacity, 2); } }
Zlib test
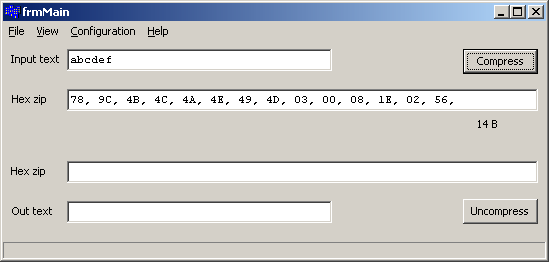
C++/CLI custom shape form (Visual C++ 2008 Express)
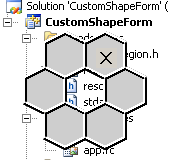
Used same hexagonal toolbar bitmap as in miniscope v4 and VCL snippet.
CustomShapeForm.zip